Okay, so I decided to make a little countdown timer for June 1st. Just something simple I wanted to see ticking away on a page.
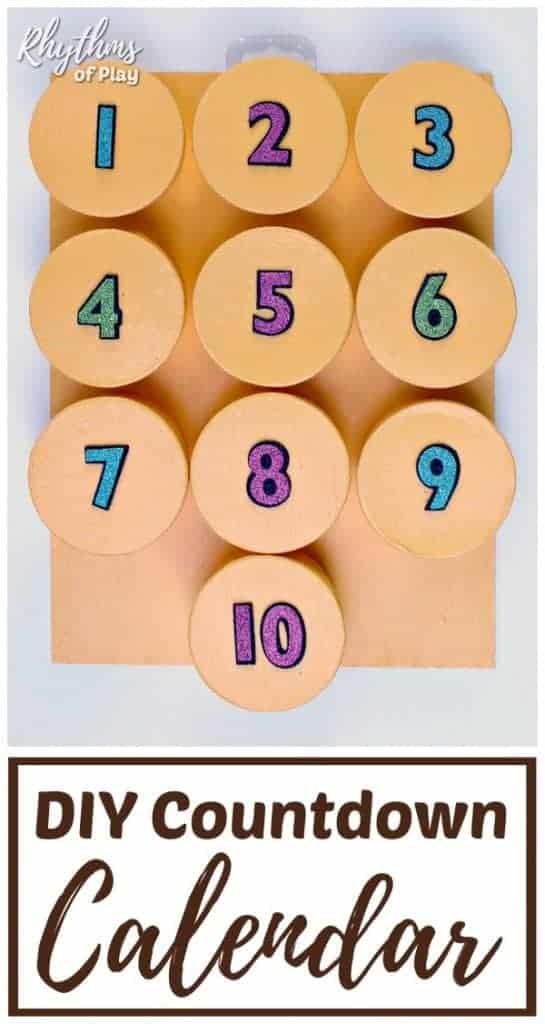
Getting Started
First thing, I just opened up my text editor. Nothing fancy. I created a basic HTML file, you know, . Just the bare bones: html, head, body tags.
Inside the body, I needed spots to show the time left. So I put in some paragraph tags, maybe gave them IDs like ‘days’, ‘hours’, ‘minutes’, ‘seconds’. Something like:
<p>Days: <strong id="days">00</strong></p>
<p>Hours: <strong id="hours">00</strong></p>
<p>Minutes: <strong id="minutes">00</strong></p>
<p>Seconds: <strong id="seconds">00</strong></p>
Super simple stuff. Then I made a file to make it look a tiny bit better. Didn’t go crazy, just centered the text on the page and maybe made the font size a bit bigger so I could see it easily.
Making it Tick
Next up was the actual countdown part. I created a file and linked it in my HTML.
Inside the script, I needed to figure out two dates: the target date (June 1st of the current year) and right now. Getting ‘now’ is easy in JavaScript. For June 1st, I just set the month and day.
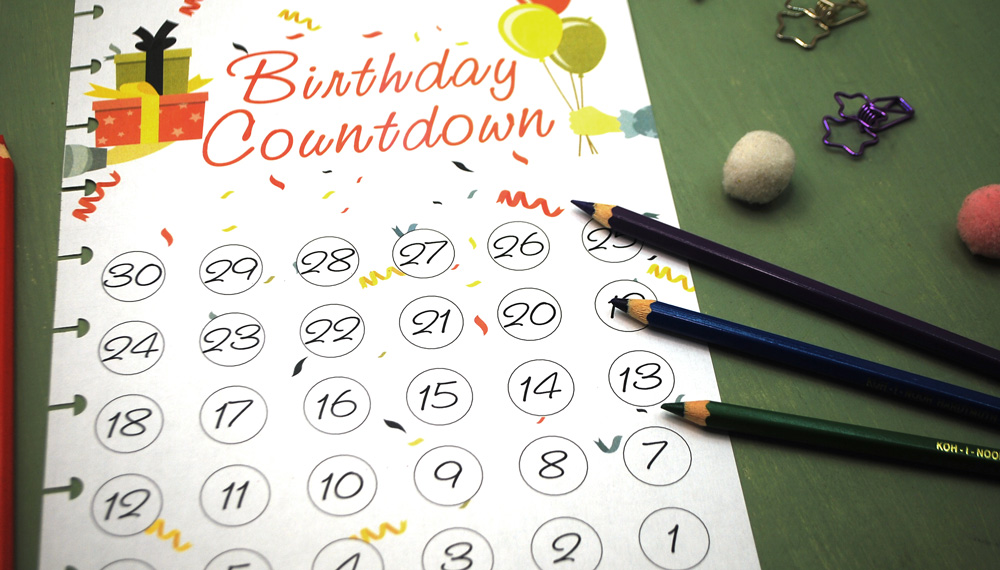
Then, the main part: calculate the difference. You subtract ‘now’ from the target date. This gives you a big number, the time difference in milliseconds. I had to remember that bit, it always trips me up if I forget it’s milliseconds.
Okay, so I had this huge number. Not very useful. I needed to break it down.
- Divided by 1000 to get seconds.
- Divided that by 60 to get minutes.
- Divided that by 60 to get hours.
- Divided that by 24 to get days.
Had to use the modulo operator (%) quite a bit here to get the remainders – like, after calculating total hours, I needed the leftover minutes, and so on. Took a few tries to get the math right, honestly. Just fiddling with the numbers until it looked correct.
Once I had the days, hours, minutes, and seconds calculated, I needed to display them. I used those IDs I set up earlier in the HTML. Grabbed each element (like `*(‘days’)`) and updated its text content with the calculated value.
Putting it on Repeat
The countdown only calculated once when the page loaded. Pretty useless. It needed to update constantly.
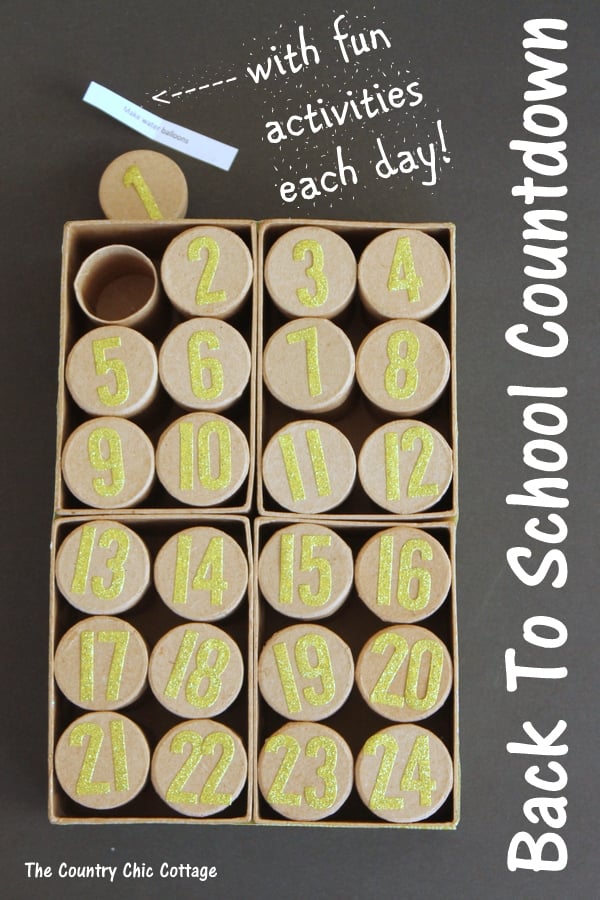
So, I wrapped all that calculation and display logic inside a function. Then I used setInterval
. Told it to run my update function every 1000 milliseconds (which is every second).
setInterval(myUpdateFunction, 1000);
That did the trick! Opened the HTML file in my browser, and boom, there it was, ticking down second by second towards June 1st. Added a little check too, so if the date passed, it would just show zeros or maybe a simple message like “It’s June 1st!”.
Wasn’t anything groundbreaking, but it was a nice little exercise. Got it done, works fine. Just a practical little thing I wanted to build and see running.