Okay, so I wanted to mess around with drawing in Python, specifically trying out this library called “hawthorn”. I’d seen some cool examples online, and figured it was time to get my hands dirty. Here’s how it went down.
Getting Started
First things first, I needed to install the thing. I fired up my terminal and typed in:
pip install hawthorn
Simple, then all l needed to do was to wait the installation to complete.
Making a Blank Canvas
Next up, I created a new Python file. I just named it “drawing_*”. Inside, I needed to import “hawthorn” and create a drawing surface.
I did the below command:
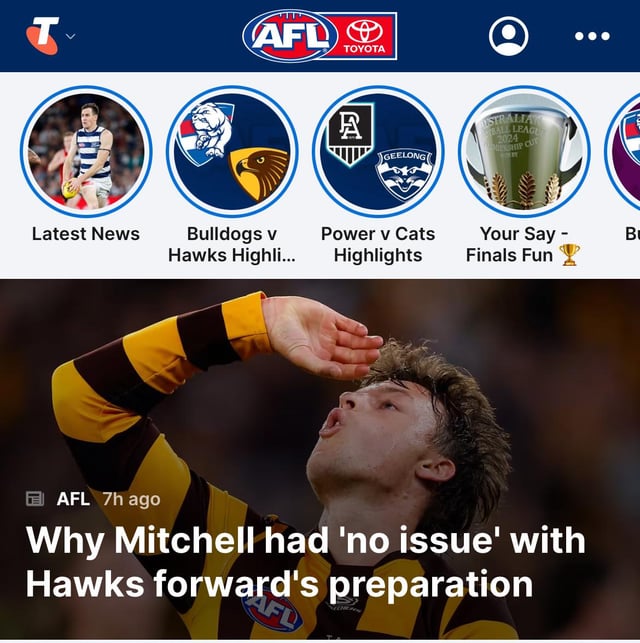
import hawthorn
surface = *_surface(800, 600)
Boom! That gave me a surface, 800 pixels wide and 600 pixels tall, stored in a variable named surface .
Drawing Something Simple
Alright, time to actually draw something. I started with a basic rectangle, nothing fancy.
I did the following:
rectangle = *(100, 50, 200, 150)
*(rectangle)
I figured, “Let’s make a rectangle. Start it at x=100, y=50, make it 200 pixels wide and 150 pixels tall.” Then, I told the surface to draw that bad boy.
Adding Some Color
A plain white rectangle? Boring. I wanted some color in my life. I needed to create a color and fill the rectangle with it.
So this is what l did:
blue = *(0, 0, 255)
*_color = blue
*(rectangle)
That’s pure blue, baby! (Red=0, Green=0, Blue=255). I set the rectangle’s fill color, and then drew it again.
Saving My Masterpiece
Finally, I wanted to save my work of art. I used *_to_png() to export it as a PNG image.
I ran:
*_to_png(“my_*”)
And that’s it, l found my_* in the folder.
The Whole Process
Putting it all together, the whole code looked like this:
- import hawthorn
- surface = *_surface(800, 600)
- rectangle = *(100, 50, 200, 150)
- blue = *(0, 0, 255)
- *_color = blue
- *(rectangle)
- *_to_png(“my_*”)
It was a pretty smooth process, all in all. I’m definitely going to play around with “hawthorn” some more and see what other shapes and effects I can create.